class: middle center # ~ Introduction to Javascript ~ --- class: middle ## Why Javascript? With HTML and CSS, the code we write is relatively static once we hit save and load it in the browser. We've learned how to add animation and limited interactivity, but even with these advance uses, our code is still fixed and only can respond in predetermined ways. --- class: middle Javascript enables you to consider a new temporal dimension of a webpage — the time before, during and after a page loads — and add dynamic and complex interactions. --- class: middle two-column With javascript we can: + add/remove classes + add/remove html elements + change the contents of elements + change the style of elements + add add mouse, keyboard, and other interactive features + load additional resources and so much
more
--- class: middle ## How to use Javascript Similar to how we use CSS, javascript can be used embedded or linked #### embedded ```html ``` #### linked ```html ``` Like with CSS, the `linked` approach is the best way to use javascript. --- class: middle fix-multiline Some basics: ```js // this is a comment! /* this is a multiline comment */ // this writes a message to the developer console // which can be useful for debugging your code! console.log('hi class~'); // a bit old school, this will create an alert box alert('hi class!!'); ``` --- class: middle Unlike HTML and CSS, Javascript is a `programming language`. Generally speaking HTML and CSS exist to express how to structure and display content. Javascript and other programming languages are like lists of instructions to be carried out or executed. Javascript is the programming language we use with web browsers, but there are [hundreds of programming languages](https://en.wikipedia.org/wiki/List_of_programming_languages) in existence. Most programming languages share a set of foundational principles. Once you understand these principles, it can be easier to learn a new programming language. --- class: middle ## How do we talk to computers? Let's go over some of these foundational principles we'll use a lot. --- class: middle #### Variables A variable is a container for a value that we can use throughout our code. We define a variable by using the `let` keyword in front of it. ```js // variables can be any name you want.. // so long as they are only made up of letters! let myVariable = 'hi'; // this won't work let my variables = 'hi'; // nor will this!! let my-variable = 'hi'; // try to avoid this let my2ndVariable = 'hi'; // this is ok, but javascript convention is to use "bumpy" case let my_variable = 'hi'; ``` --- class: middle Variables can hold lots of different values.. like `strings` and `numbers`: ```js let myNumber = 10; // we can use console.log to see the variable in the console console.log(myNumber); let anotherVariable = 'hi'; console.log(anotherVariable); // we can reuse a variable once we've created it! // note that we don't re-define it with `let` before // since it already exists anotherVariable = 'ho'; console.log(anotherVariable); // here we're adding together the string "hi" to // our `anotherVariable` that is equal to "ho" let offToWorkWeGo = 'hi ' + anotherVariable; console.log(offToWorkWeGo); ``` --- class: middle #### Conditionals A `conditional` is a way that we can ask a question and do different things based on the answer. Conditionals are written with an **`if` statement** with the question wrapped in parentheses `()` and the code to run if the question is true in `{ ... }` curly brackets: ```js let name = 'goose'; if (name == 'goose') { console.log('run!!!'); } ``` Often we use conditionals to compare one value to another. The way we compare values is with `Comparison Operators`. Above we're using the `==` comparator, which asks is the value on the left is the same as the value on the right. --- class: middle two-column Other common comparison operators are: + `==` is exactly equal + `!=` is not exactly euqal + `>` is greater than + `<` is less than + `>=` is greater than or equal + `<=` is less than or equal --- class: middle Extending our last example, let's also add a condition for when the item isn't true. ```js let name = 'duck'; if (name == 'goose') { console.log('run!!!'); } else { console.log('whew!'); } ``` Here we and an `else` condition, for when the first conditional isn't true. --- class: middle fix-multiline fix-again-ugh There's a third way to control the flow of your conditionals, with the **`else if` statement**: ```js /* eggs: 0 0 0 0 0 0 0 0 0 0 0 0 */ let guess = 8; // how many eggs do we have? if (guess < 12) { console.log("you're under!"); } else if (guess > 12) { console.log("you're over!"); } else if (guess == 12) { console.log("bingo!"); } ``` This allows you to chain together multiple conditionals or questions in order to handle more cases. --- class: middle #### A quick note about spacing The formatting of certain items in javascript is flexible: ```js // this is valid if(1==1){ console.log('yeah, good. OK.'); } ``` But certain conventions have been established to ensure things remain readable and consistent. The convention for writing conditionals is to put a space after the `if`, around operators, and before the curly: ```js if (1 == 1) { ``` This isn't a necessity, but a good habit to get into.
: )
--- class: middle There are several other common programming principles.
We'll cover those in the coming weeks~ --- class: middle The `DOM` = `D`ocument `O`bject `M`odel .tree-img[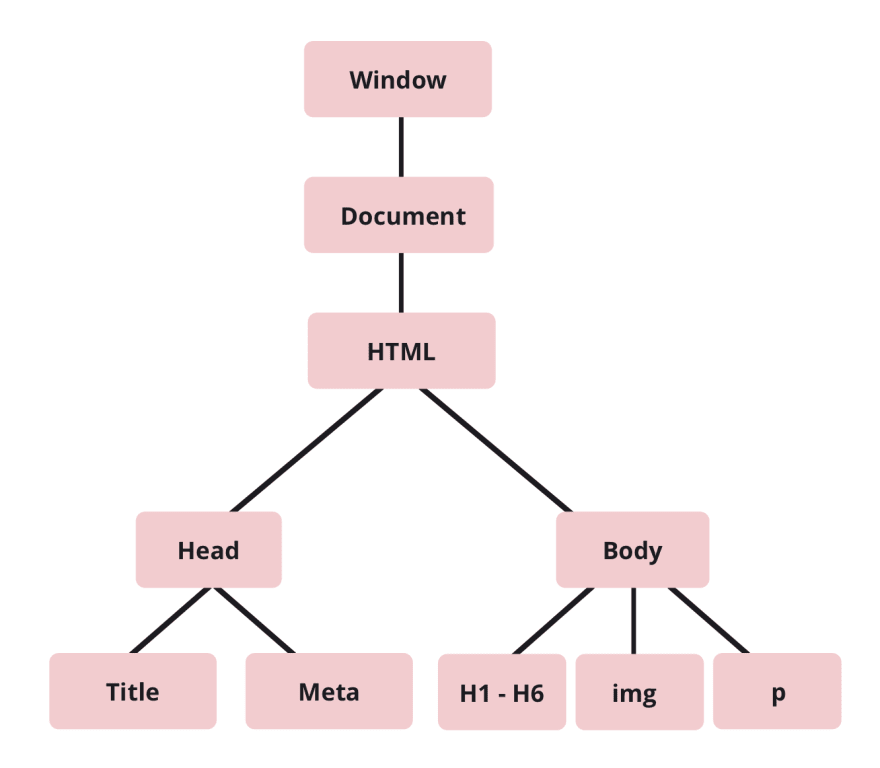] .footnote[diagram from https://dev.to/karaluton/what-exactly-is-the-dom-jhg] --- class: middle One of the most common uses for javascript is to make changes to the `DOM`. --- class: middle background-image: url(assets/domtree.png) background-size: 100% ### Plucking elements from the `DOM` tree In order to make changes to an element, we first need to select it in javascript. The way to target elements in javascript is with the `querySelector` method. --- class: middle example.js: ```js let blogElement = document.querySelector('#blog'); console.log(blogElement); ``` example.html: ```html
``` ...wait why does it say `null`? `null` is a value that is returned When something isn't found.. --- class: middle Since our javascript is executed line by line as the html document is `parsed` the `#blog` div doesn't exist at the time the `querySelector` line is executed.. The document parsed these lines one by one ```html
``` and then moved to our js file: ```js let blogElement = document.querySelector('#blog'); console.log(blogElement); ``` --- class: middle There are two common solutions to this problem... either move your code that selects elements after those elements have been parsed: ```html
```
--- class: middle Or more simply, add the `defer` attribute to your script tag to tell the browser to apply your Javascript code after the page has been completely parsed. ```html
``` **note** this will only work for externally loaded files. Embded javascript, you'll need to place the script tag after the body or use other techniques we'll learn more about later.. --- class: middle example.js: ```js let blogElement = document.querySelector('#blog'); console.log(blogElement); ``` example.html: ```html
hello class
```
hello class
--- class: middle Now that we have a refrence to our element in javascript, we can do something with it like adding a class: ```css .red { color: red; } ``` The `classList` attribute of a html element in javascript provides tools for adding, removing, and checking whether an element has a class. ```js let blogElement = document.querySelector('#blog'); blogElement.classList.add('red'); ```
hello class